class, 객체, 생성자
- 객체를 생성할 때, 생성자를 넣지 않는 경우 undefined로 출력
- 객체 생성 후, 객체 설정 추가가능! (굳이 생성자를 자세히 만들 필요 X)
- 생성자는 자바스크립트 하나에 오직 하나만 허용!!
예시
- 예) 은행 - 입금/출금/이체 https://jungeun980906.tistory.com/214
- 예) 생성자 함수이용 클래스 생성
생성
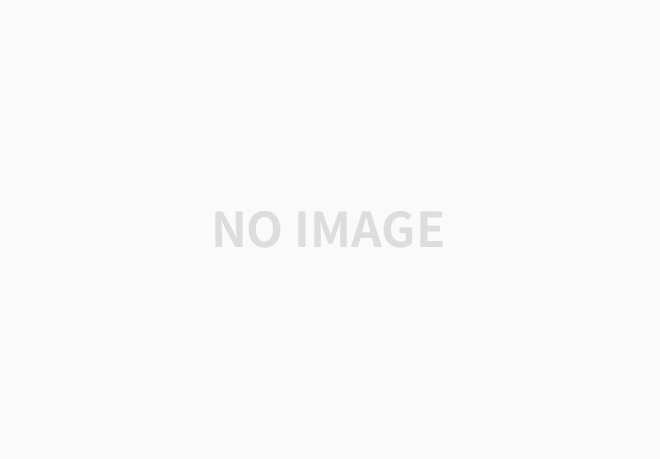
<script>
// 클래스생성
class Car{
// 생성자 생성
: 자바스크립트에서는 생성자는 오직 1개! 허용
constructor (name, year){
this.name = name;
this.year = year;
this.model = '';
}
}
// 객체생성
let myCar1 = new Car('쏘나타', 2022);
let myCar2 = new Car('그랜저', 2022);
let myCar3 = new Car();
myCar3.name = '아반떼';
myCar3.year = 2001;
myCar3.model = 'XD';
let myCar4 = new Car('부릉부릉');
myCar4.year = 2023;
console.log(myCar1);
console.log(myCar2);
console.log(myCar3);
console.log(myCar4);
console.log(myCar4.name); // 속성: name 이름만 출력
console.log(myCar4.year); // 속성: year 연식만 출력
</script>
생성 기본문법
- 클래스 명은 대문자 시작
- set : 보통 알림창을 띄우는 용도로 사용
class MyClass {
// 생성자
constructor( ) {
...
}
//메서드 정의
method1( ) { ... }
method2( ) { ... }
method3( ) { ... }
//getter, setter
get name( ){
return this.name;
}
set name(value){
if(value.length < 4){
alter('길이가 4글자 이상이여야합니다');
return;
}
this.name = value;
}
}
// 사용
let user = New MyClass(param) ;
생성자 함수
- 생성자 함수는 클래스의 함수화 한 것
- 일반함수 = 생성자함수의 기술적 차이
- 단,
- 생성자 함수는 반드시 new를 이용하여 실행해야함!
- 생성자 함수 첫 글자는 대문자
- get / set 사용 불가
function User(name){
this.name = name;
this.age = 0;
}
let user = new User('정은;);
console.log(user.name); // 정은
user.age = 26;
console.log(user.age); // 26
생성 (클래스->객체로 생성, 생성자 함수로 생성, 리터럴 객체로 생성)
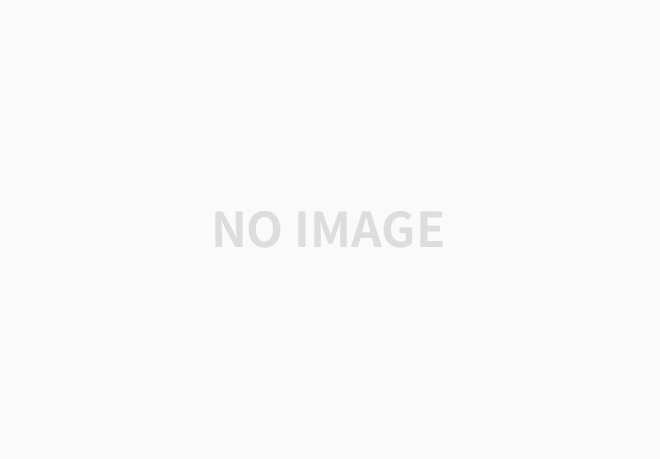
<script>
//클래스 생성 - 리터럴 객체(비어있는객체) 생성 - 생성자 함수 생성
//정의할 프로퍼티(멤버변수) : firstName., lastName, age, address
//메서드 : 함수를 호출하면 프로퍼티의 값이 출력 (toString같은 개념)
class Make{
// 생성자
constructor(firstName,lastName,age,address){
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
this.address = address;
}
//출력메서드
toString(){
// console.log(this.firstName,this.lastName,this.age,this.address);
return `${this.firstName}, ${this.lastName}, ${this.age}, ${this.address}`;
}
//get
get toString1(){
return `${this.firstName}, ${this.lastName}, ${this.age}, ${this.address}`;
}
}
//class로 객체생성, 출력
let human1 = new Make('박','정은',26,'인천');
//human1.toString(); //사용가능
console.log(human1); //Make
console.log(human1.toString()); //메서드는 ()괄호 (박,정은,26,인천)
console.log(human1.toString1); //get은 이름만 (박,정은,26,인천)
console.log('-------------------------------');
//----------------------------------------------------------------------------------------------
//생성자 함수 사용
//생성자함수 (get X)
function User(firstName, lastName, age, address){
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
this.address = address;
this.print1 = function(){
// console.log(this.firstName,this.lastName,this.age,this.address);
return `${this.firstName}, ${this.lastName}, ${this.age}, ${this.address}`;
}
}
//생성자함수로 생성, 출력
let human2 = new User('밥','다래','1','영국');
// human2.print1(); //사용가능 (밥 다래 1 영국)
console.log(human2); //User
console.log(human2.print1()); // (밥, 다래, 1, 영국)
console.log('-------------------------------');
//-------------------------------------------------------------------------------------------------
//리터럴 객체 사용
const person = {
firstName: "",
lastName: "",
age: "",
address: "",
//set함수
setInfo: function(firstName,lastName,age,address){
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
this.address = address;
},
//get
get personInfo(){
return `${this.firstName}, ${this.lastName}, ${this.age}, ${this.address}`;
},
//set
set setFirstName(first){
this.firstName = first;
}
}
person.setInfo('박','희은',23,'수원');
console.log(person); //setInfo 출력
</script>
'HTML.CSS.JS > JS' 카테고리의 다른 글
[JS] lotto번호 생성 / 출력 (set, [ ] 이용) (0) | 2023.04.18 |
---|---|
[JS] class 예제 : 은행 (입금, 출금, 이체) (0) | 2023.04.18 |
[JS] Map 예제 : 과목, 점수를 map에 저장하고 합계,평균 등 출력 (0) | 2023.04.18 |
[JS] Count버튼 만들기 (더하기버튼누르면 +1/ 뺴기버튼누르면 -1) (0) | 2023.04.18 |
[JS] try~catch 예외 처리 (0) | 2023.04.18 |